In this guide, we’ll walk you through creating a simple ESP32 Web Server that displays “Hello, World!” on a webpage. The ESP32 microcontroller is a fantastic platform for IoT projects, and one of its standout features is its ability to host a webserver.
What is a web server?
A webserver is computer software and underlying hardware that accepts requests via HTTP (the network protocol created to distribute web content) or its secure variant HTTPS.
If you want to know more about a web server, you can visit Webserver – Wikipedia
What You’ll Need
- ESP32 development board
- USB cable for connection
- Arduino IDE installed and configured for ESP32 (How To Install ESP32 And ESP8266 Boards In Arduino IDE (Step-by-Step Guide) – ArduinoYard)
- Wi-Fi credentials (SSID and password)
Step 1: Install Required Libraries
Ensure you have the latest version of the ESPAsyncWebServer and AsyncTCP libraries installed:
- Go to Sketch > Include Library > Manage Libraries in the Arduino IDE.
- Search for ESPAsyncWebServer and install it.
- Search for AsyncTCP and install it.
Step 2: Connect the ESP32
- Connect your ESP32 board to your computer via USB.
- Open Arduino IDE and select the appropriate board (e.g., “ESP32 Dev Module”) and port from the Tools menu.
Step 3: Write the Code
Below is the code to create a simple ESP32 web server:
// ESP32 Web Server: Hello World!
#include <WiFi.h>
#include <ESPAsyncWebServer.h>
// Replace with your network credentials
const char* ssid = "YourWiFiSSID";
const char* password = "YourWiFiPass";
// Create a web server object on port 80
AsyncWebServer server(80);
// HTML webpage with inline CSS styling for icons and layout
const char index_html[] PROGMEM = R"rawliteral(
<!DOCTYPE html>
<html lang="en">
<body>
Hello,world!
</body>
</html>
)rawliteral";
void setup() {
Serial.begin(9600);
// Connect to Wi-Fi
Serial.println("Connecting to Wi-Fi...");
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.print(".");
}
Serial.println("\nWi-Fi connected!");
Serial.println("IP Address: ");
Serial.println(WiFi.localIP());
// Define the root URL handler
server.on("/", HTTP_GET, [](AsyncWebServerRequest *request) {
request->send(200, "text/html", index_html);
});
// Start the server
server.begin();
Serial.println("Web server started.");
}
void loop() {
}
Step 4: Upload the Code
- Copy and paste the above code into the Arduino IDE.
- Replace
Your_SSID
andYour_PASSWORD
with your Wi-Fi network credentials. - Click the Upload button.
- Open the Serial Monitor (baud rate 9600) to view the ESP32’s IP address.
Step 5: Access the Web Server
- After uploading, open the Serial Monitor to find the ESP32’s IP address (e.g.,
192.168.
xx.xx). - Enter the IP address in a web browser on the same Wi-Fi network.
- You should see a simple webpage displaying Hello, World!
Expected Output
Serial Monitor Output:
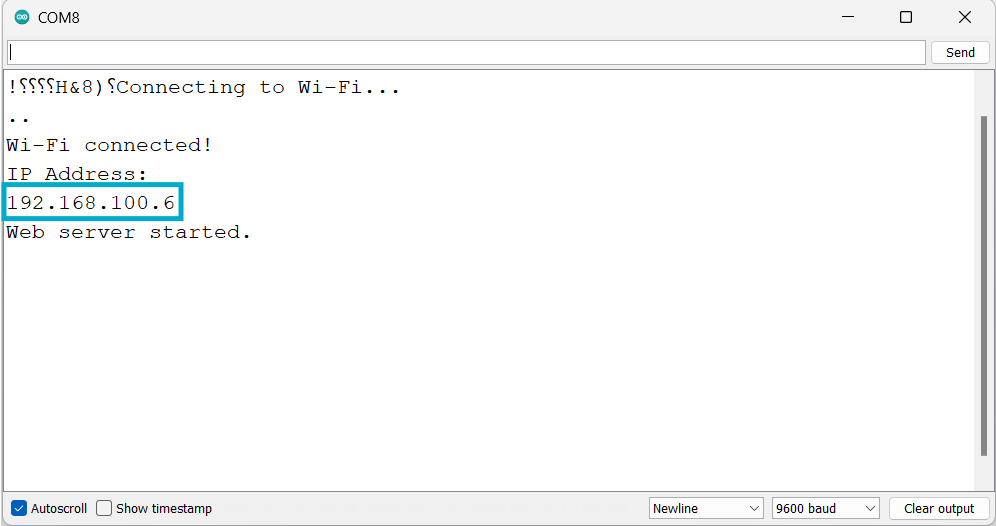
Browser Output:
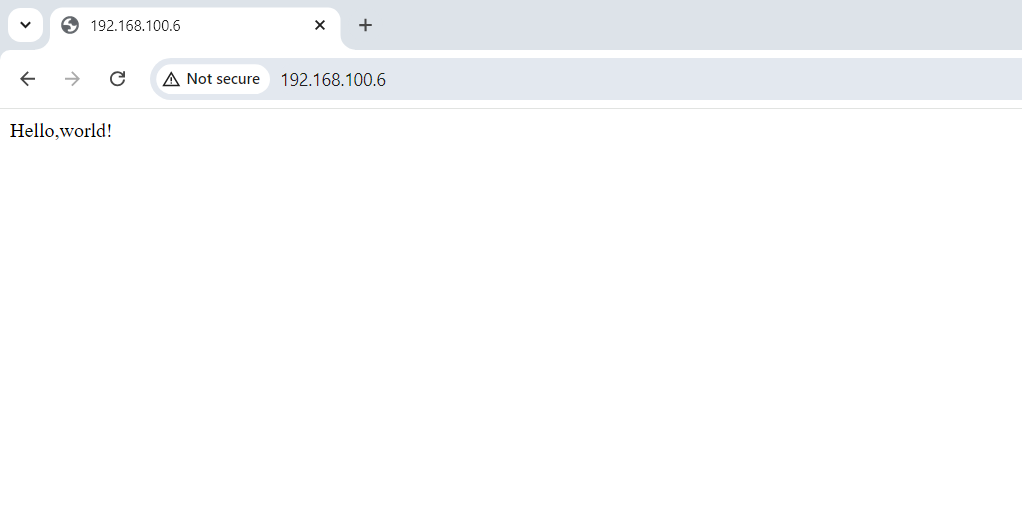
How It Works
- Wi-Fi Connection: The ESP32 connects to your Wi-Fi using the
WiFi.begin()
method. - Web Server: The
ESPAsyncWebServer
library hosts a simple server on port 80. - URL Handler: When the browser accesses the root URL (
/
), the server sends a response with “Hello, World!” as plain text.
Common Issues
- Cannot Connect to Wi-Fi: Ensure you entered the correct SSID and password.
- No Response in Browser: Verify that the browser and ESP32 are on the same network.
- Upload Errors: Press and hold the “BOOT” button during upload if necessary.
Applications
Once you’ve created this simple server, you can expand it to:
- Display sensor data
- Control devices remotely
- Create interactive IoT dashboards
Congratulations! You’ve successfully created a simple ESP32 web server. In the next tutorial we will show you how can we create a button to control an LED with the ESP32 web server. This “Hello, World!” example is just the beginning of the amazing IoT projects you can build. Happy coding!
If you are interested in amazing esp32 projects, you can visit our website.
Web Based Weather Station With ESP32 And DHT11 Sensor – ArduinoYard
DIY Home Security: Arduino Intruder Alarm System With ESP32 & Blynk Cloud Integration – ArduinoYard
YouTube Channel Statistics Display With NodeMCU(ESP8266) And I2C OLED Display – Easy – ArduinoYard