Table of Contents
Overview
Discover how to kickstart your OLED display projects with Arduino! Learn step-by-step guidance, wiring, and code example to get started with I2C OLED Display with Arduino effortlessly.
Read about OLED displays: https://www.oled-info.com/oled-introduction
Wiring the OLED Display to Arduino
To connect an OLED display to an Arduino, you will need an OLED display module, such as the 0.96 inch OLED display module, and an Arduino board, such as the Arduino Uno.
You will also need some wires to connect the OLED display module to the Arduino board.
Here’s how to connect the OLED display module to the Arduino Uno:
Connect the OLED display module’s VCC pin to the Arduino Uno’s 5V pin
Connect the OLED display module’s GND pin to the Arduino Uno’s GND pin
Connect the OLED display module’s SDA pin to the Arduino Uno’s A4 pin
Connect the OLED display module’s SCL pin to the Arduino Uno’s A5 pin
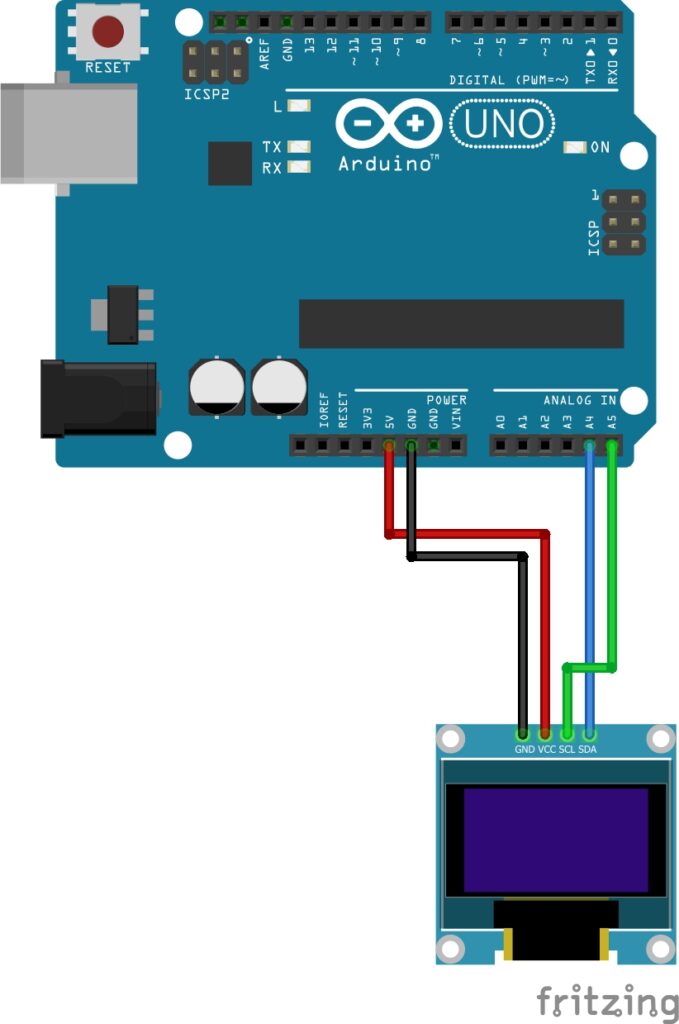
Installing the Required Libraries
In Arduino IDE, Go to sketch-> Include library->Library manager-> Search for Adafruit_GFX and Adafruit_SSD1306 libraries to install them.
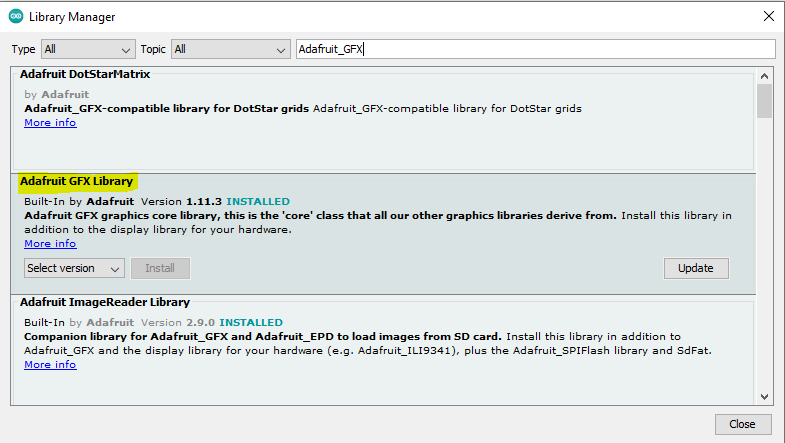

Programming the OLED
To program the OLED display with Arduino, you will need to use a library that supports OLED displays. There are several libraries available for OLED displays, such as the Adafruit SSD1306 library.
Here’s an example code for displaying “Hello, World!” on the OLED display using the Adafruit SSD1306 library:
// I2C OLED Display with Arduino by ArduinoYard.com
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define OLED_RESET 4
Adafruit_SSD1306 display(OLED_RESET);
void setup() {
display.begin(SSD1306_SWITCHCAPVCC, 0x3C);
display.display();
delay(2000);
}
void loop() {
display.clearDisplay();
display.setTextColor(WHITE);
display.setTextSize(2);
display.setCursor(0, 10);
display.println("Hello,");
display.setCursor(0, 30);
display.println("World!");
display.display();
delay(2000);
}
This code uses the Adafruit SSD1306 library to display “Hello, World!” on the OLED display. The setup() function initializes the OLED display, and the loop() function clears the display and prints the message “Hello, World!” every 2 seconds.
Possible Issues
If you’re having issues with the address of your OLED display, it’s likely because the display is using a different address than the one specified in your code.
Most OLED displays use either the SSD1306 or SH1106 driver chip, which have different I2C addresses.
The SSD1306 typically uses the address 0x3C, while the SH1106 uses the address 0x3D. However, some displays may use different addresses, depending on the manufacturer or model.
To resolve the address issue, you can try the following steps:
Check the datasheet or product description of your OLED display to see if it specifies the I2C address. Use an I2C scanner sketch to find the correct address.
An I2C scanner sketch is a simple Arduino program that scans all the I2C addresses on the bus and reports which ones are being used. Here’s an example I2C scanner sketch:
#include <Wire.h>
void setup() {
Wire.begin();
Serial.begin(9600);
while (!Serial); // Wait for serial monitor to open
Serial.println("\nI2C Scanner");
}
void loop() {
byte error, address;
int nDevices;
Serial.println("Scanning…");
nDevices = 0;
for (address = 1; address < 127; address++ )
{
Wire.beginTransmission(address);
error = Wire.endTransmission();
if (error == 0)
{
Serial.print("I2C device found at address 0x");
if (address < 16)
Serial.print("0");
Serial.print(address, HEX);
Serial.println(" !");
nDevices++;
}
else if (error == 4)
{
Serial.print("Unknow error at address 0x");
if (address < 16)
Serial.print("0");
Serial.println(address, HEX);
}
}
if (nDevices == 0)
Serial.println("No I2C devices found\n");
else
Serial.println("Done\n");
delay(5000); // Wait 5 seconds before scanning again
}
Once you upload this sketch to your Arduino board and open the serial monitor, you should see a list of all the I2C devices on the bus, along with their addresses. Look for an address that matches your OLED display.
Demonstration
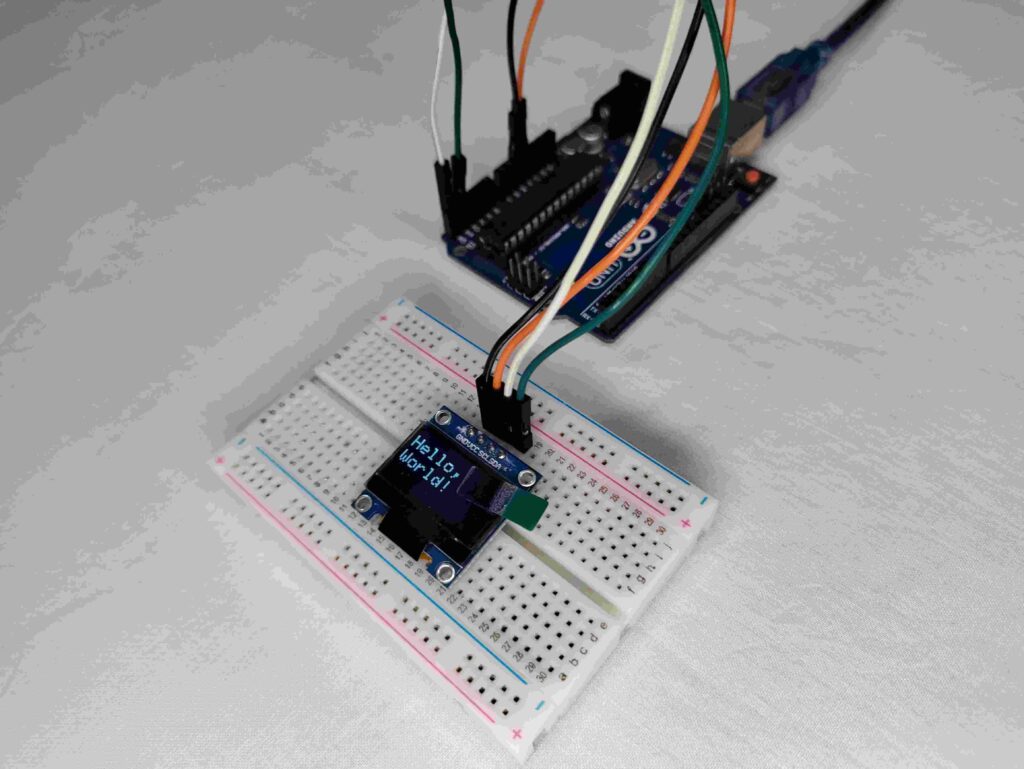
Conclusion
OLED displays are a versatile and powerful tool for displaying information in your Arduino projects.
By following this beginner’s guide, you should be able to connect an OLED display to an Arduino and display text or graphics on it using a library like the Adafruit SSD1306 library.
With some creativity and experimentation, you can use OLED displays to create unique and innovative projects like:
YOUTUBE CHANNEL STATISTICS DISPLAY WITH NODEMCU(ESP8266) AND I2C OLED DISPLAY
Thank you!