Introduction
In this guide, we will cover how to use the the MQ-5 Gas Sensor with Arduino, and build projects to detect and display gas concentration levels.
The MQ-5 gas sensor is widely used for detecting LPG (Liquefied Petroleum Gas), natural gas, and town gas leaks. It operates by measuring changes in resistance when exposed to combustible gases. This makes it ideal for gas leak detection in kitchens, factories, and industrial applications.
Understanding the MQ-5 Gas Sensor
The MQ-5 gas sensor is a metal oxide semiconductor (MOS) sensor that operates based on changes in resistance. The sensor consists of a heating element and a chemically sensitive layer (SnO2 – tin dioxide). When combustible gases come into contact with the sensor, the resistance of the sensing element changes, which alters the output voltage.
How It Works
- The sensor has a built-in heater that maintains an optimal operating temperature.
- When LPG, methane, or natural gas is present, it interacts with the SnO2 layer, reducing its resistance.
- This change in resistance is converted into a voltage output.
- Higher gas concentration results in a higher output voltage.
- The analog output (A0) provides a variable voltage proportional to gas concentration.
- The digital output (D0) is triggered when the gas level exceeds a preset threshold (adjustable via the onboard potentiometer).
Key Features of MQ-5
- Operating Voltage: 5V
- Gas Detection: LPG, methane, natural gas
- Preheat Time: At least 24 hours for accurate readings
- Analog Output: Provides varying voltage based on gas concentration
- Digital Output: Turns HIGH when the gas level crosses a threshold
- Long lifespan and high sensitivity
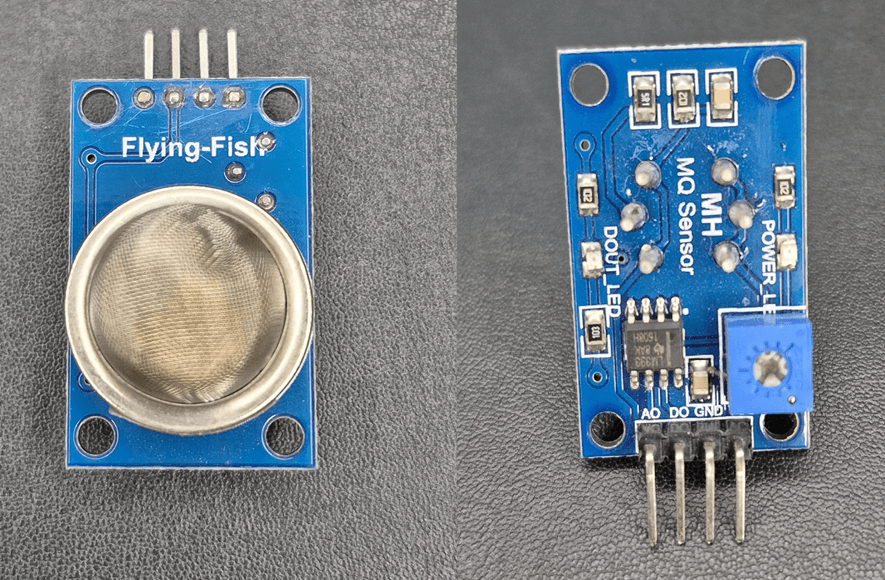
Required Components
- Arduino Uno/Nano
- MQ-5 Gas Sensor Module
- Buzzer
- LED (Optional for visual alert)
- Jumper Wires
MQ-5 Gas Sensor with Arduino Connections
MQ-5 Pin | Description | Arduino Connection |
---|---|---|
VCC | Power Supply (5V) | 5V |
GND | Ground | GND |
A0 | Analog Output | A0 |
D0 | Digital Output | Any Digital Pin (D2) |
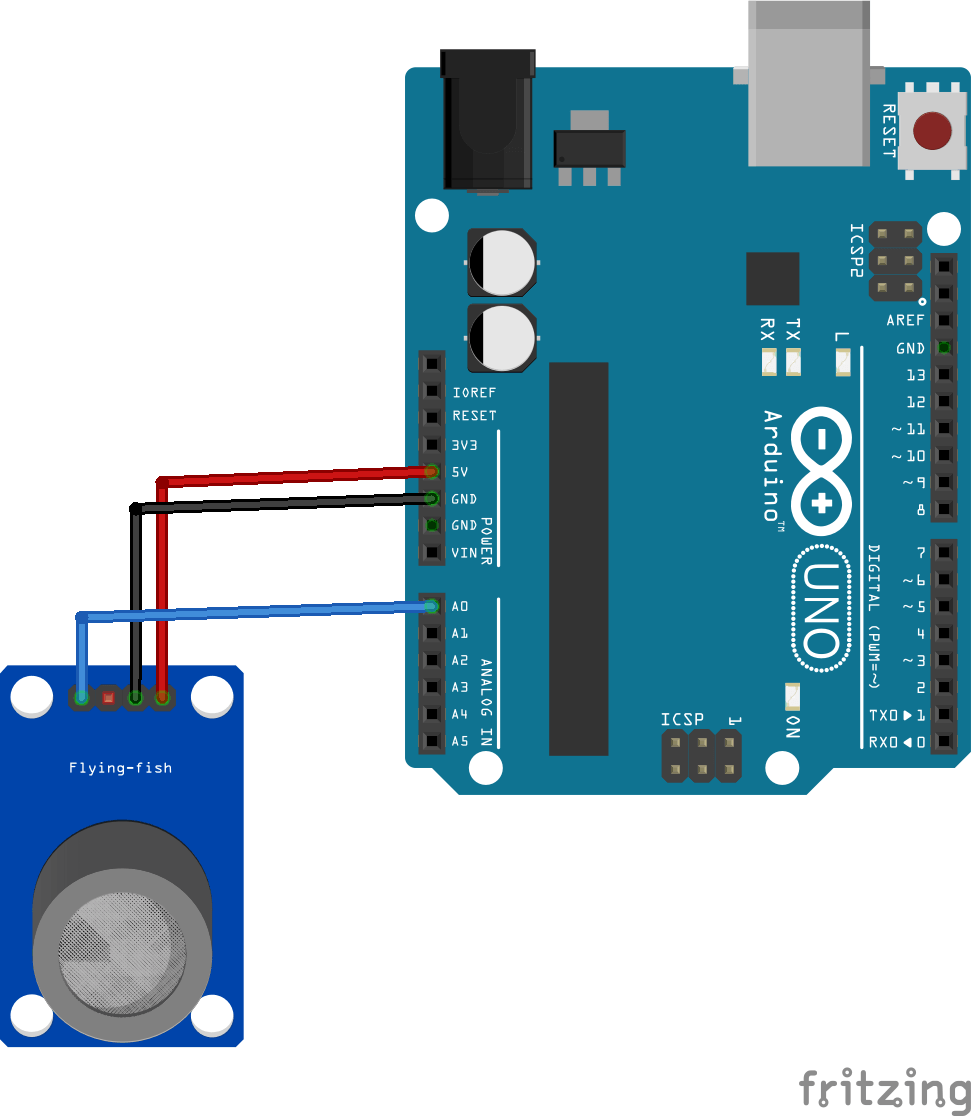
Basic Code to Read Gas Levels from MQ-5
The following code reads gas concentration levels from the MQ-5 sensor and prints them to the Serial Monitor.
// MQ-5 Gas Sensor with Arduino by ArduinoYard
#define MQ5_PIN A0 // Define the analog pin for MQ-5
void setup() {
Serial.begin(9600); // Start Serial communication
}
void loop() {
int sensorValue = analogRead(MQ5_PIN); // Read gas sensor value
Serial.print("Gas Level: ");
Serial.println(sensorValue); // Print value to Serial Monitor
delay(1000); // Wait for a second before next reading
}
Hardware:
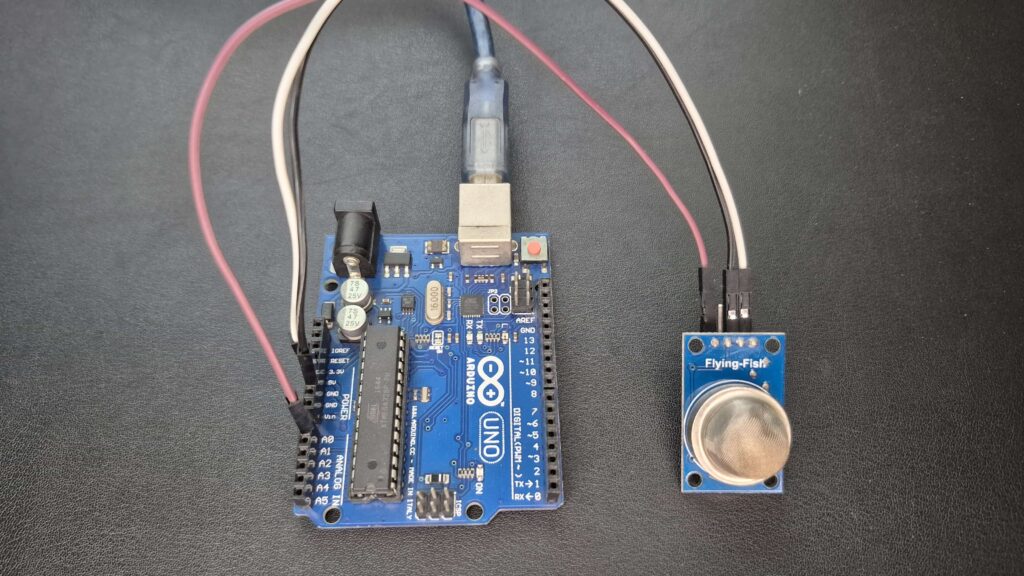
Serial Monitor:
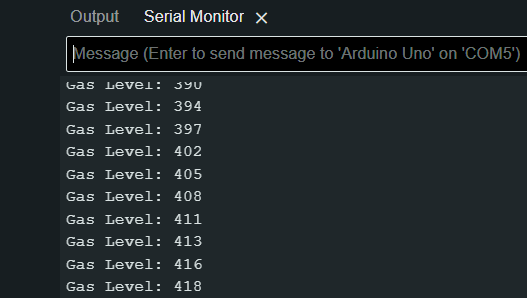
Gas Leak Detection with Buzzer Alert
Wiring:
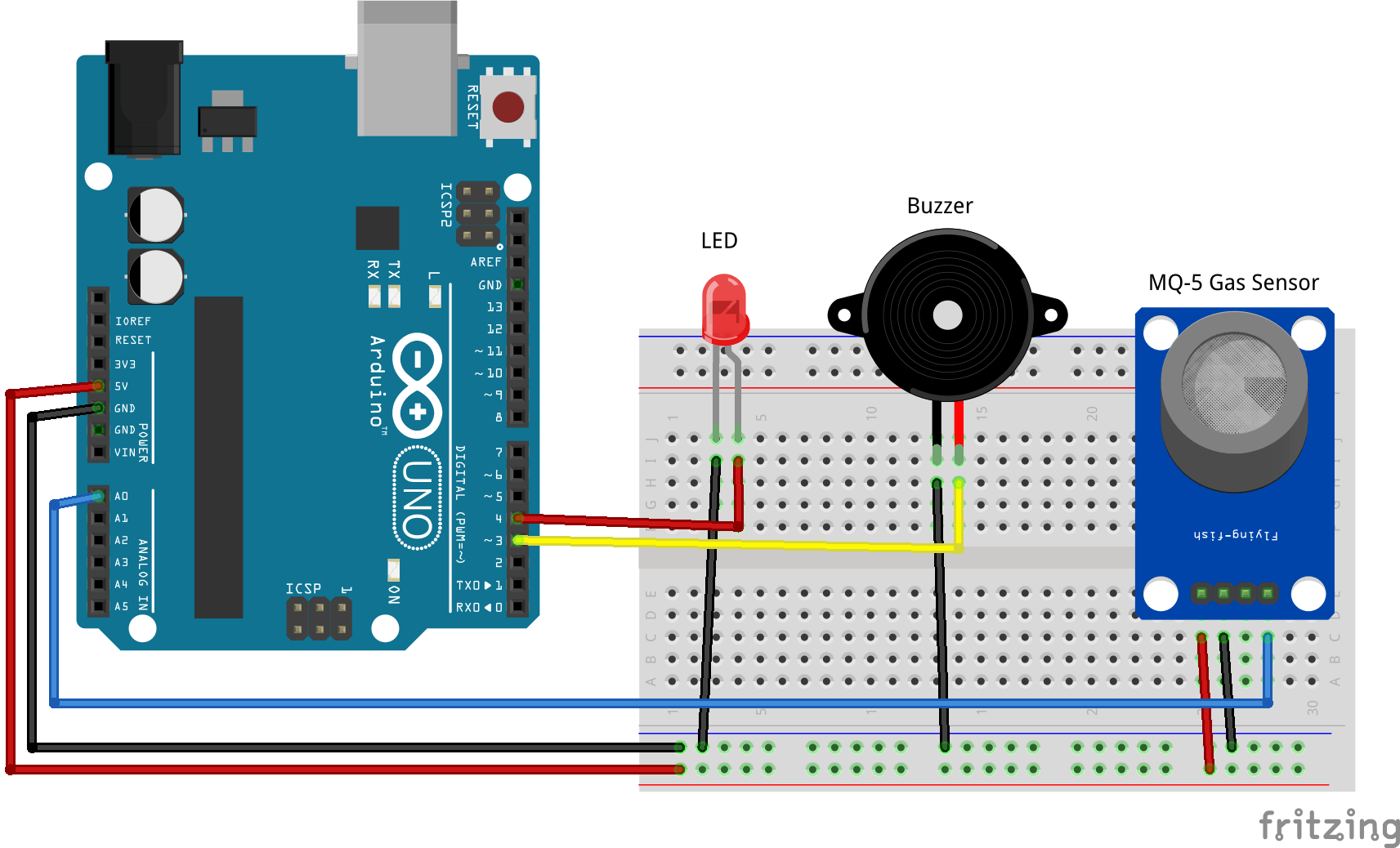
Code:
This code triggers a buzzer when gas levels exceed a set threshold, indicating a potential gas leak.
// MQ-5 Gas Sensor with Arduino by ArduinoYard
#define MQ5_PIN A0 // MQ-5 sensor analog output pin
#define BUZZER_PIN 3 // Buzzer connected to digital pin 3
#define LED_PIN 4 // LED connected to digital pin 4
void setup() {
Serial.begin(9600);
pinMode(LED_PIN, OUTPUT); // Set LED pin as output
}
void loop() {
int gasLevel = analogRead(MQ5_PIN); // Read gas level from MQ-5 sensor
Serial.print("Gas Level: ");
Serial.println(gasLevel);
if (gasLevel > 500) { // Threshold for gas detection
digitalWrite(LED_PIN, HIGH); // Turn on LED
tone(BUZZER_PIN, 1000); // Play tone at 1000 Hz
Serial.println("Gas Leak Detected!");
} else {
digitalWrite(LED_PIN, LOW); // Turn off LED
noTone(BUZZER_PIN); // Stop buzzer
}
delay(1000); // Wait for 1 second before the next reading
}
Applications and Project Ideas
The MQ-5 gas sensor is used in various applications, including:
- Gas Leakage Detection Systems – Automatic gas leak detection in homes and industries.
- Smart Gas Monitoring Systems – Wireless gas level monitoring with alerts.
- Industrial Safety Systems – Preventing hazardous gas leaks in factories.
- Automated Gas Stove Shut-off – Turning off gas supply in case of leaks.
Project Ideas:
- Gas Leak Detector with GSM Alert – Sends an SMS alert when gas levels are high.
- WiFi-Based Gas Monitoring System – Logs data to an IoT dashboard.
- Fire Alarm System – Triggers an alarm when gas concentration is high.
- Car Cabin Air Quality Monitor – Checks for harmful gases inside vehicles.
Useful Links
Conclusion
In this guide, we explored how to interface the MQ-5 Gas Sensor with Arduino, understand its working, and implement gas leak detection projects. With its ability to detect LPG, methane, and natural gas, the MQ-5 sensor is a valuable tool for safety applications.
Have any questions? Let us know in the comments! 🚀