Introduction
Infrared (IR) sensors are widely used in electronics for detecting objects and measuring distance. They operate by emitting infrared light and detecting its reflection from nearby objects. These sensors are especially popular in projects like obstacle detection, line-following robots, and automation systems. In this article, we’ll explore the basics of using an IR Sensor with Arduino and create a simple project to demonstrate its functionality: turning an LED ON or OFF based on the proximity of an obstacle.
Understanding the IR Sensor Module
The IR sensor module typically consists of two main components:
- IR Emitter (LED): Emits infrared light.
- IR Receiver (Photodiode): Detects the reflected infrared light when it hits an obstacle.
The module also includes supporting circuitry, such as:
- A comparator to provide a digital output based on the intensity of reflected light.
- A potentiometer to adjust the sensitivity of the sensor.
Pinout of a Typical IR Sensor Module
Here is the pinout of an IR sensor:
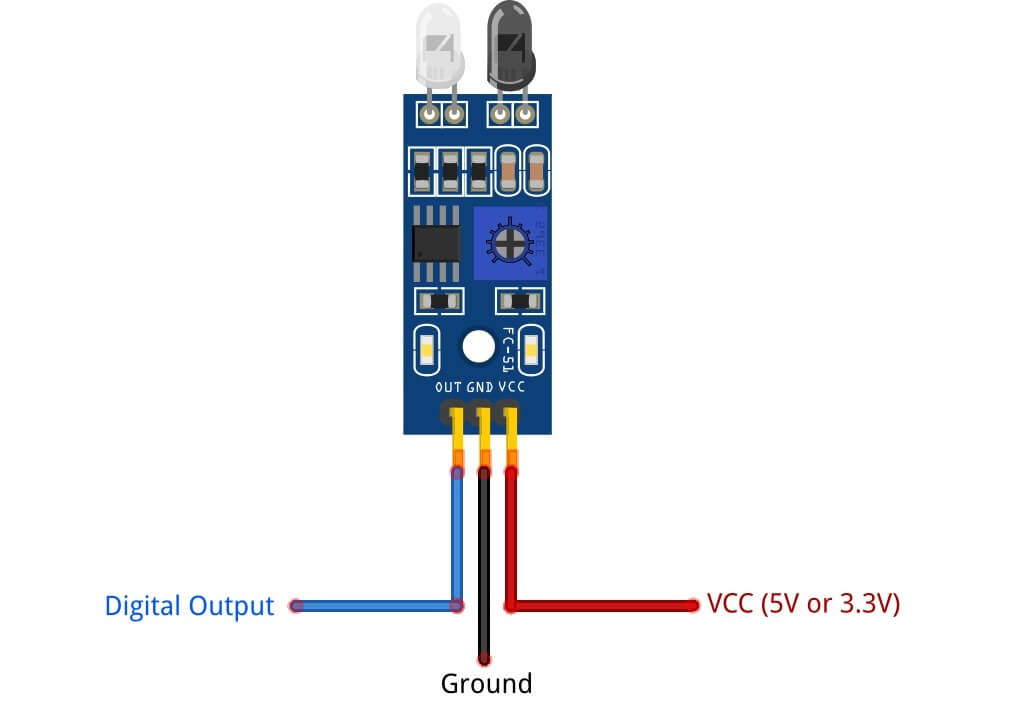
- VCC: Connects to a 5V or 3.3V or a microcontroller or a power supply.
- GND: Connects to ground.
- OUT: Digital output that goes HIGH or LOW based on obstacle detection.
How the IR Sensor Works
- The IR LED continuously emits infrared light.
- When an object comes into the range of the sensor, the infrared light reflects off the object and is detected by the photodiode.
- The module processes this reflected light and outputs a HIGH or LOW signal to indicate the presence of an object.
Learn more about Infrared: Wikipedia – Infrared
Learn more about Infrared Sensors/Modules: Understanding IR Sensors and IR LEDs
Components Required
- Arduino Uno (or any other compatible board)
- IR Sensor Module
- LED
- 220-ohm Resistor
- Breadboard and Jumper Wires
Wiring the IR Sensor with Arduino
Here’s how to connect the IR Sensor with Arduino and an LED:
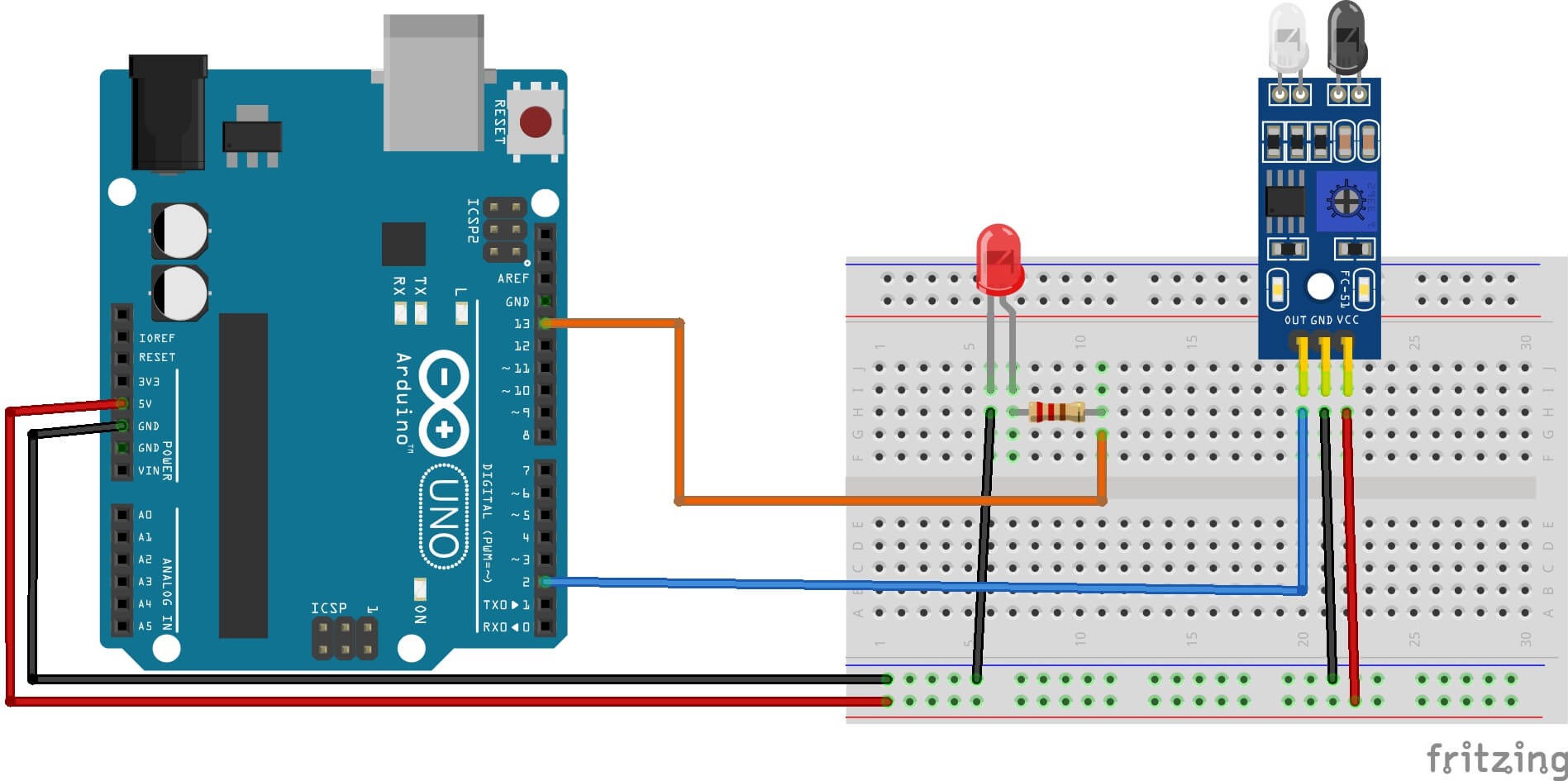
- IR Sensor Module:
- VCC to Arduino 5V
- GND to Arduino GND
- OUT to Arduino digital pin 2
- LED Circuit:
- Positive leg (anode) of the LED to Arduino digital pin 13 (via a 220-ohm resistor).
- Negative leg (cathode) of the LED to Arduino GND.
Arduino Code for the IR Sensor Project
The following code turns the LED ON when an obstacle is detected and OFF when there is no obstacle:
// Using IR Sensor with Arduino (Proximity Sensor) - Complete Guide by ArduinoYard
// Pin Definitions
const int irSensorPin = 2; // IR Sensor Output
const int ledPin = 13; // LED Pin
void setup() {
pinMode(irSensorPin, INPUT); // Set IR sensor pin as input
pinMode(ledPin, OUTPUT); // Set LED pin as output
Serial.begin(9600); // Initialize serial communication
}
void loop() {
int sensorValue = digitalRead(irSensorPin); // Read the IR sensor output
if (sensorValue == LOW) { // If Obstacle detected (This might be HIGH for your sensor, based on whether it is active-low or active-high. Adjust if required.)
digitalWrite(ledPin, HIGH);
Serial.println("Obstacle detected!");
} else { // No obstacle
digitalWrite(ledPin, LOW);
Serial.println("No obstacle.");
}
delay(100); // Small delay for stability
}
How the Code Works
- The irSensorPin is read using
digitalRead()
. If it returnsHIGH
, it means an obstacle is detected. - The ledPin is controlled using
digitalWrite()
. When an obstacle is detected, the LED is turned ON; otherwise, it remains OFF. - Serial messages are printed to the Serial Monitor for real-time feedback.
Testing the Project
- Upload the code to your Arduino board.
- Open the Serial Monitor to observe the sensor readings.
- Place an object in front of the IR sensor to see the LED turn ON and the message “Obstacle detected!” appear in the Serial Monitor.
- Remove the object, and the LED will turn OFF with the message “No obstacle.”
Serial Monitor:

Demonstration:
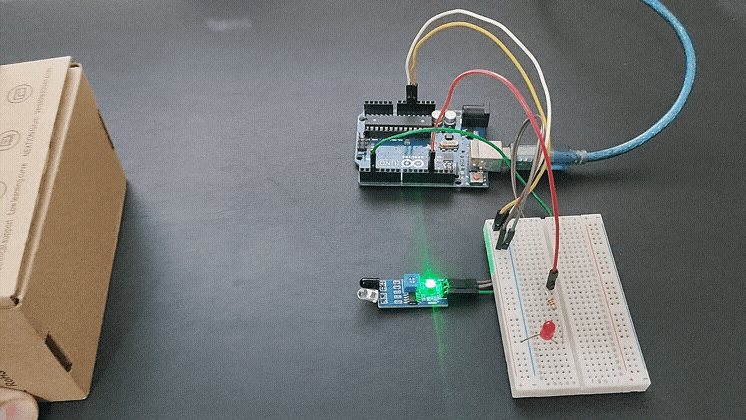
Troubleshooting Tips
- Sensitivity Adjustment: Use the onboard potentiometer to adjust the detection range.
- Ambient Light Interference: IR sensors may be affected by sunlight or other strong light sources. Test your setup in a controlled environment.
- Stable Connections: Ensure all connections are secure and there are no loose wires.
Conclusion
Using an IR Sensor with Arduino is a straightforward way to create efficient object detection systems. With just a few components, you can implement exciting proximity-based projects. This simple LED ON/OFF project demonstrates the basic working of an IR sensor module, and you can expand this concept into more complex projects like automated systems or robotics.
Applications of IR Sensor with Arduino
- Obstacle detection in robots
- Automatic door opening systems
- Line-following robots
- Safety systems for machinery
These versatile sensors open the door to endless possibilities for DIY and professional electronics projects.
If you’re excited to explore more complex applications of IR sensors, check out this related project on Autonomous Edge Detection Robot with Arduino. This project demonstrates the advanced use of IR sensor with Arduino in robotics, helping you take your skills to the next level.
Explore more Arduino projects and tutorials on ArduinoYard to take your skills to the next level!